Este é um exemplo de como trabalhar com JSON em C#.
E com jQuery vou mostrar como consumir o webservice que vai retornar o JSON.
Abaixo fiz um formulário com os campos "Palavra" e "Número de repetições".
Estes dados serão enviados para um web service via jQuery.
Este web service vai retornar um JSON com as palavras repetidas conforme os parâmetros passados.
Na verdade é um exemplo bem simples, mas podemos ver:
Como transformar um objeto em JSON usando o JavaScriptSerializer e como fazer um webservice retornar JSON.
JavaScriptSerializer é suportado nas versões: 4.5, 4, 3.5 do .NET Framework.
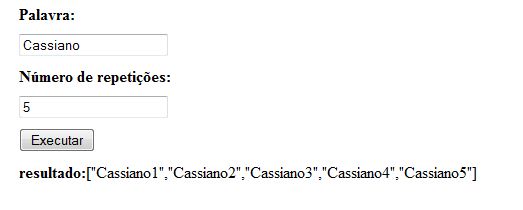
App_Code/webservice.cs
using System;
using System.Collections;
using System.Linq;
using System.Web;
using System.Web.Services;
using System.Web.Services.Protocols;
using System.Xml.Linq;
using System.Data.SqlClient;
using System.Data;
using System.Diagnostics;
using System.Web.Script.Services;
using System.Web.Script.Serialization;
using System.Collections.Generic;
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
// To allow this Web Service to be called from script, using ASP.NET AJAX, uncomment the following line.
[System.Web.Script.Services.ScriptService]
public class webservice : System.Web.Services.WebService
{
//Retorna o formato JSON
[ScriptMethod(ResponseFormat = ResponseFormat.Json)]
[WebMethod()]
public string RetornaJSON(string word, string n)
{
//Lista de palavras
List<string> list = new List<string>();
//Criando a lista de palavras
for (int i = 1; i <= Convert.ToInt32(n); i++)
{
list.Add(word + i);
}
//O JavaScriptSerializer vai fazer o web service retornar JSON
JavaScriptSerializer js = new JavaScriptSerializer();
//Converte e retorna os dados em JSON
return js.Serialize(list);
}
}
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<script src="/jquery-1.8.1.min.js"></script>
<script type="text/javascript">
function GetData() {
$.ajax({
type: 'POST'
//Caminho do WebService + / + nome do metodo
, url: "/webservice.asmx/RetornaJSON"
, contentType: 'application/json; charset=utf-8'
, dataType: 'json'
//Adicionando a palavra e o número de repetições.
//, data: "{word:'Cassiano', n:'5'}"
, data: "{word:'" + escape($("#palavra").val()) + "', n:'" + $("#n").val() + "'}"
, success: function (data, status) {
//Tratando o retorno com parseJSON
var itens = $.parseJSON(data.d);
//Alert com o primeiro item
alert(itens[0]);
//Respondendo na tela todos os itens
$('.resultado').text(data.d);
}
, error: function (xmlHttpRequest, status, err) {
//Caso ocorra algum erro:
$('.resultado').html('Ocorreu um erro');
}
});
}
</script>
</head>
<body>
<form id="form1" runat="server">
<div>
<div>
<strong>Palavra:</strong>
</div>
<div>
<input type="text" id="palavra" />
</div>
<div>
<strong>Número de repetições:</strong>
</div>
<div>
<input type="text" id="n" />
</div>
<div>
<input type="button" onclick="GetData();" value="Executar" />
</div>
<div>
<strong>resultado:</strong><span class="resultado">Null</span>
</div>
</div>
</form>
</body>
</html>
Faça download do exemplo completo aqui.